Google Address Validation API
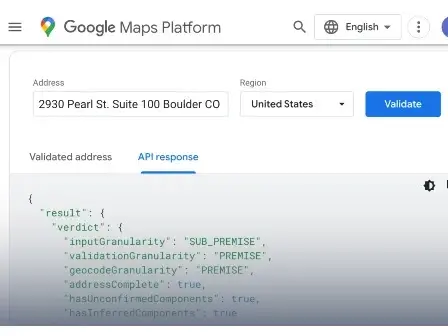
The Google Address Validation API is an address verification tool launched under the Google Maps Platform which parses and standardizes addresses and compares them to official datasets to determine if the address is real. The API works in over 30 countries, and it provides valuable information like geocodes and RDI for many addresses.
Smarty™ also offers address validation APIs for US and International addresses. Smarty's address validation APIs are lightning-fast, well-documented, backed by our legendary support, have few data analysis and storage restrictions, and validates addresses in over 240 countries.
Smarty's address validation tool will help you and your business to save money, company time, and any frustrations encountered by returned mail and invalid address information within your address database. Try out our tools, or read through our documentation here:
US Address Validation API | International Address Validation API | API Documentation |
---|---|---|
In this article, we'll cover:
- What is Google's Address Validation API?
- Who is Google's Address Validation API for?
- How to Use Google's Address Validation API (Python Example)
- Google Address Validation API Limitations
- How Smarty's Address Validation API Compares
What is Google's Address Validation API?
Google's Address Validation API parses addresses into their components and standardizes them to meet the standards of the local postal authority like USPS for US addresses. The verification API also confirms if an entered mailing address exists and includes all necessary components, such as unit numbers and ZIP+4 Codes. This API is accessible through the Google Maps Platform suite of APIs in their Places product category.
Key Features
Feature | Description and Function |
Parsing | It breaks addresses into components but doesn't always correctly separate the Address Line 1 components (e.g., N (north)) and suffix components (e.g., St. (street) from other parts of the address |
Primary Data Sources | USPS, local postal authorities, Google Maps Platform's Places |
CASS Certified | References the United States Postal Service®'s Coding Accuracy Support System (CASSTM) |
International Address Verification | It has a single API for approximately 33 countries |
Residential Delivery Indicator (RDI) | Though the tool provides RDI data for a few countries, the data is not always accurate |
API Architectural Styles | REST and gRPC |
Query Rate Limit | 100 Queries Per Second (QPS) |
Batch Data Processing | Requires custom code to send each item in a batch as a separate request |
Geocoding | Provides both geocoding location data and specific address information |
Autocomplete Address | Yes, but it doesn't include validation/standardization and suggests non-existent/incomplete addresses |
3rd-Party Compatibility | Yes, but it may be limited by terms of use & licensing restrictions |
Pricing
To use Google's Address Validation API, users must pay based on SKU usage and the price per use. Google offsets the cost of using its address validation tool by providing all users $200 worth of free credit per month. This credit amounts to almost 12,000 address validation lookups or is usable for any other Maps, Routes, and Places products. Additional Address Validation API requests start at around 58 requests/$1, and Google offers high-volume pricing discounts.
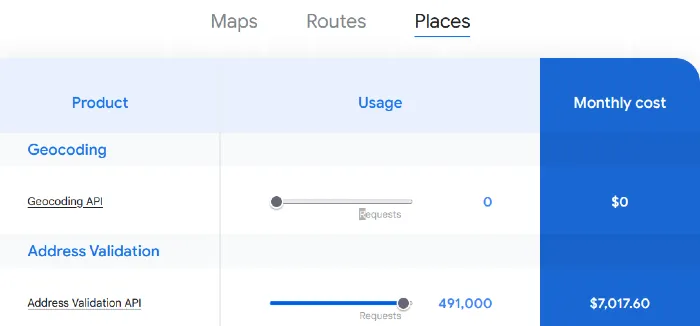
Terms of Use - Restrictions
Though this list is far from exhaustive, it includes some critical restrictions to consider before integration. You can read the full Terms of Use here.
Data Caching: Most address data can't be stored beyond 30 days. The exception to this rule is if the data is needed for a "downstream transaction," such as a quarterly subscription for supplements.
Displaying Validated Address: Users are not allowed to display Address Validation API data on any map other than Google Maps.
Data Analysis: Data from Google's Address Validation API can't be used for analysis.
Attribution Requirements: Any application that displays data from Google Maps' Address Validation API, must display Google's logo.

Who is Google's Address Validation API for?
Benefits of Address Validation for Businesses and Developers
There are many ways to save on business costs with Address Validation Software, such as:
Reduces Shipping Costs: When you ship to the wrong address, you lose the money you spent for the packaging, shipping, and personnel labor that went into preparing and sending the package. The USPS also charges fees for rerouting a package or returning the package to the sender.
Protects Against Fraud: Sending mail to the wrong address can open both your business and your customers to potential fraud, especially if the mail is confidential. Individuals could also use alias addresses to sign up for multiple one-time offers and free trials.
Better Customer Service: Having accurate addresses eliminates problems for you, your customers, and the post office.
More Marketing Opportunities: Ensuring your direct mail reaches the right people increases the number of people you can reach.
Use Case for Google's Address Validation API
Small eCommerce companies are the most viable users for Google Maps' Address Validation API. Smaller companies can take full advantage of the free $200 in credit. As long as they remain under approximately 12,000 address validation lookups a month, the tool is completely free to use.
Though the API doesn't check addresses for every country, having a single tool for both international and US addresses can save businesses time. Even deleting the data cache every 30 days is less of a hassle for small eCommerce companies if they do not have frequent repeat clients.
Though eCommerce companies can generally get by with just Google Maps Address Validation API, the address verification tool lacks the necessary functionality for many businesses. Because of the strict Terms of Use, companies will need a more robust tool for location data intelligence, enrichment, bending, analysis, and cleansing. Even the free $200 becomes insignificant; those credits are used up fast, considering companies often need to rerun queries through multiple APIs because of Google's strict data retention policies.
How to Use Google's Address Validation API - Python Example
Enable Google Address Validation API
-
In the Google Cloud Platform console, select a project (or create a new one), and then select the Menu..
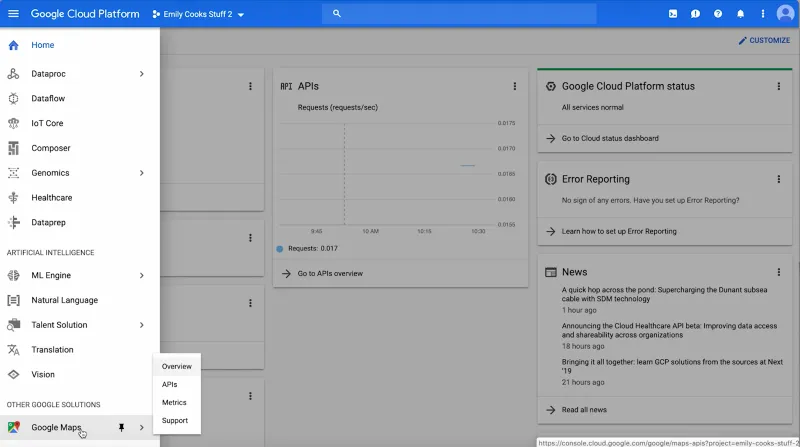
-
In the menu, hover over Google Maps and then select APIs.
-
From the dashboard, select Google Address Validation API. (Depending on your previous activity, at this point, you might be asked for billing information and to answer a questionnaire before you can move on to the next steps.)
-
From the API overview page, select Enable.
Create a Google Address Validation API Key
-
In the Google Cloud Platform console, select a project, and then select the Menu.
-
In the menu, hover over APIs & Services and select Credentials.
-
From the Credentials page, select Create Credentials -> API Key. Your API key will appear in a popup box.
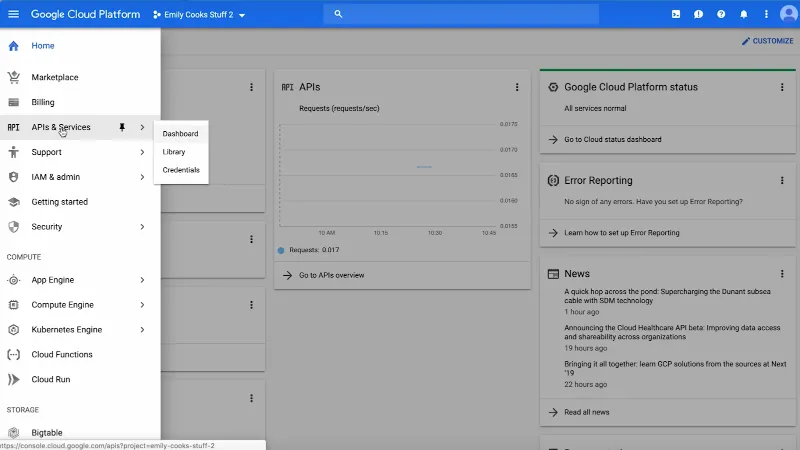
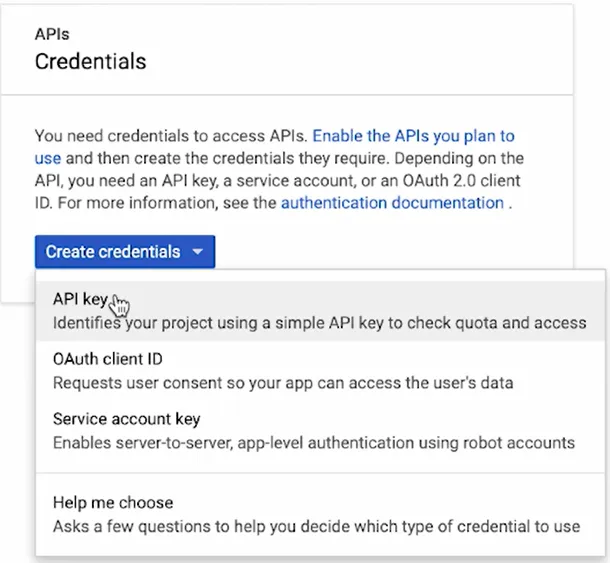
Python Program
This code uses an environment variable GOOGLE_API_KEY that's set to the API Key we just created. Run the following Python program to access the Google Address Validation API. The below example validates an address in Canada.
Before you start your Python adventure, here are a couple of tips to help you on your journey:
-
import requests
- This command imports the "requests" library. You'll need to have the library installed for this to work. Luckily, installing the library is easy. Open your command line and run the command pip install requests OR python -m pip install requests. Either command will install the requests package through the Python package manager. -
googleKey = os.getenv('GOOGLE_API_KEY')
- this code uses an environment variable os.getenv('GOOGLE_API_KEY'). Using environment variables for sensitive information (like API keys) is a best practice to prevent malicious use when your code inevitably ends up in a public repository or gets exposed in some other way. Environment variables require additional configuration on your machine; the steps change depending on your machine's OS. Learn how to configure them on Windows, macOS, or Linux.
If you're just testing things out you and don't want to bother with the hassle of configuring environment variables, you can enter your API key directly by updating the code from:
googleKey = os.getenv('GOOGLE_API_KEY')
To:googleKey = "your google api key here"
Then, paste your actual API key between the quotes. Remember to return and replace it once you get beyond the testing phase.
Now, onto the Python:
# python 3
import requests
import os
def run():
googleKey = os.getenv('GOOGLE_API_KEY')
payload = {
"address": {
"regionCode": "CA", # must be a two character country code
"addressLines": ["1201 Ave Van Horne"],
"locality": "Outremont",
"administrativeArea": "QC",
"postalCode": "H2V 1K4"
},
"enableUspsCass": False # set to True for US addresses
}
url = "https://addressvalidation.googleapis.com/v1:validateAddress?key=" + googleKey
response = requests.post(url, json=payload)
if response.status_code != 200:
print("Error making HTTP call:")
print(response.text)
exit()
# print the entire json response
print(response.text)
# select elements of the response
responseJson = response.json()
location = responseJson['result']['geocode']['location']
print("Address: " + responseJson['result']['address']['formattedAddress'])
print("Geocode: " + str(location['latitude']) + ", " + str(location['longitude']))
if __name__ == "__main__":
run()
This program will print both selected elements of the response (e.g., formatted address and geocode) and the entire JSON response.
Where to Use Google's Address Validation API
You can easily incorporate the Google Maps API into nearly any software system, including websites, mobile device apps, browser extensions, CRMs, etc. This means that you can integrate an Address Validation API into any of your existing systems that use address data. Commonly, companies use Address Validation API for checkout forms and employee or customer online accounts.
-
Identify where you want to integrate the Google Address Validation API. Options include web applications, mobile applications, and backend systems.
Google Address Validation API Limitations
Google's API comes with some great features but also has some limitations that may be deal breakers for your needs. Finding out before you're waist-deep in code with a looming deadline is probably better. Here are a few of the limitations you may encounter.
High Risk of False Positives
Google Maps' Address Validation API tries to answer every query, even if the entry is incoherent. Because of this, the address validation tool will sometimes take overly extreme measures to find matches for the postal address. When an address validation tool incorrectly matches a user entry with the wrong address, it creates a "false-positive." A false positive address looks like a valid address but isn't the address intended by the user.
Consider the following example:
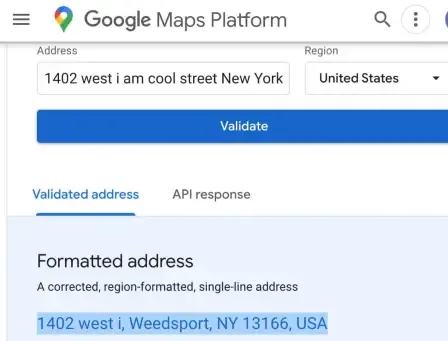
Because "1402 west i am cool street New York" doesn't exist, the address validation API didn't identify the address. Instead, they tried to auto-correct the words in the query as if the address was simply spelled blatantly wrong.
If companies aren't aware of how much the user entry is changed during validation, packages get shipped to these "false positive" addresses instead of their intended target.
Inconsistent Address Parsing
Considering address parsing is an essential part of address validation, bad parsing capabilities limit the usefulness of address verification tools. When Google's API for address validation parses a US postal address, it separates the individual address components by house number, unit designator, street name, city, state, ZIP+4 Code, and country.
However, doing so groups street types and suffix directions with the street name.
For example, entering "218 N Main St Payson Utah" in Google Maps' Address Validation API, the address validation tool labels the address components as follows:
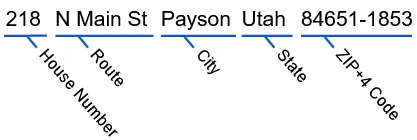
Other Address Validation APIs can parse addresses on a more granular level, as follows:
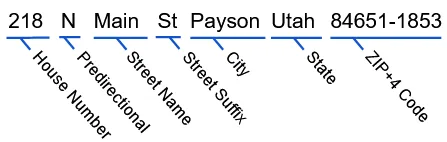
The United States Postal Service records have over 200 valid street suffixes, and Google's Address Validation API sometimes confuses these with other words or abbreviations in the street name. In this case, "St" could mean "Street" or "Saint." This ambiguity can lead to costly shipping and data errors.
Inconsistent RDI Data
Shipping rates differ for residential and commercial addresses, so correct RDI data is needed to determine accurate shipping pricing. Though Google's Address Validation API provides RDI data, the data is sometimes inaccurate or missing.
Speed
High-volume and bulk address validation options are limited to only 100 queries per second. If you were validating every address in the US, it'd take more than 3 weeks running 24/7 at full capacity with Google to complete the task. Other providers (like Smarty) can do the same work in less than 45 minutes with processing speeds of up to 75,000+ queries per second.
Non-Postal Support
In the US, more than 20 million valid, deliverable addresses aren't present in the USPS database. Google's address validation API relies heavily on USPS data, so it struggles to validate the 10%+ of the US addresses not found in the US postal service's dataset. How would a 10% address validation failure rate impact your implementation?
Cumbersome Bulk Validation
Google Maps Platform's Address Validation API is designed to accept addresses one at a time which works best when incorporated into user-facing and other single-entry address forms. When companies realize they need validation, they almost always have a large backlog of address data that also requires parsing, standardization, and validation too.
To process large datasets with the Google Places Address Validation API, you'll need to write custom code to manage the sending, receiving, and organizing of all those single address requests and extends the process of getting it into production.
We'll cover Google's restrictive data storage terms next, as it also severely impacts those needing high-volume address validation since the data can only be stored beyond 30 days if the address is necessary for a "downstream transaction."
Restrictive Data Storage Terms
Google severely limits data usage obtained from their address validation API. Google states that you can't "pre-fetch, index, store, or cache any Content" with limited exceptions. Such strict data storage terms can cause:
- Re-entering queries monthly for repeat customers.
- Reduced or eliminated the ability to track, analyze, maintain, and understand user data.
- Preventing you from collecting essential business data like user location and which service area/ franchise location they reside in.
- Costing employee time and labor to ensure that your company complies with Google's policies.
Here's the message from their policy page:

And here's the only exception the company offers on the Google Maps Platform Service Terms page:
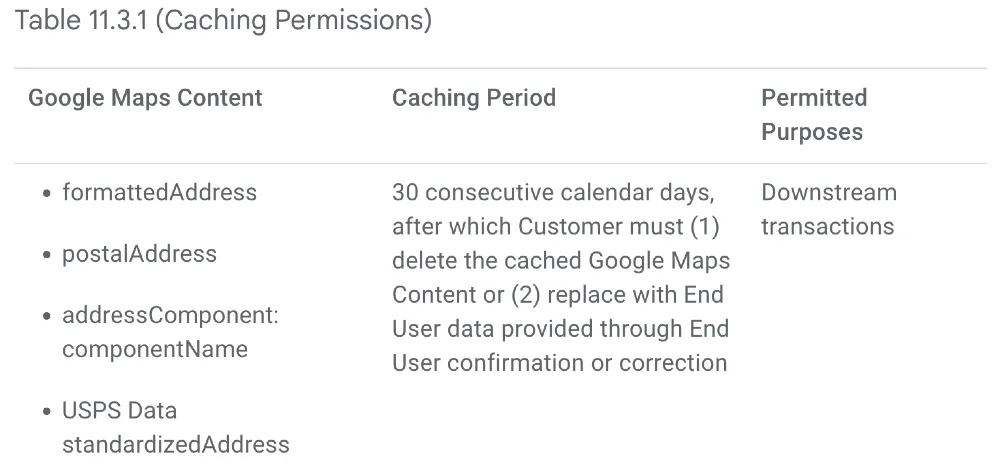
If you're handling address data for any other purpose than a "downstream transaction", you're not permitted to store the address data at all. Full stop.
Strict Attribution Requirements
Unless the address data shows on the same screen as a Google Map, anywhere Google's data appears must also display an unaltered Google Logo. Furthermore, Google has strict requirements for placement requirements for attribution.
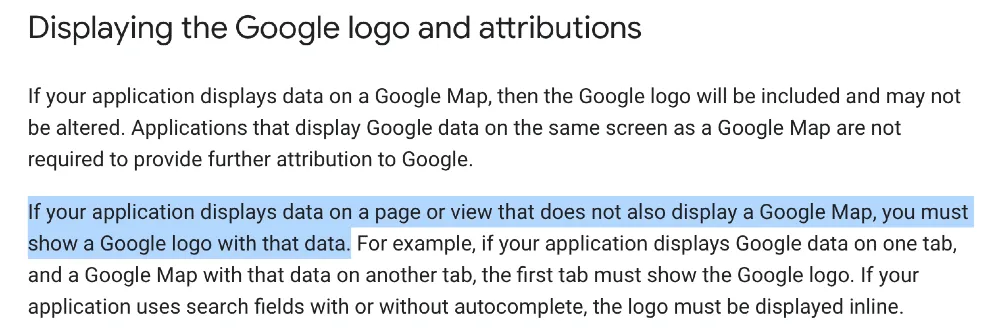
Invalid Address Autocomplete Suggestions
Google's Address Autocomplete API can be used to help customers complete location fields during checkout on an eCommerce site. When address validation is built directly into an autocomplete API, a single API can suggest exclusively valid addresses. Autocomplete and validation built into the same API reduces checkout friction, speeds up address entry, and improves data quality without sacrificing user experience.
However, Google's Address Autocomplete API predates their validation API by over a decade. So, their autocomplete suggestion data comes from unvalidated, unstandardized, crowd-sourced datasets that contain invalid, incomplete, and even entirely fake addresses.
Google suggests using the autocomplete API to autofill addresses and their validation API to correct the invalid addresses that the autocomplete API suggests. This approach leads to multiple API calls for every address, adds friction address entry forms, and introduces more opportunities for entry errors.
For example, a search for this "100000% Fake" address with Google's Address Autocomplete API shows nothing but invalid address suggestions:
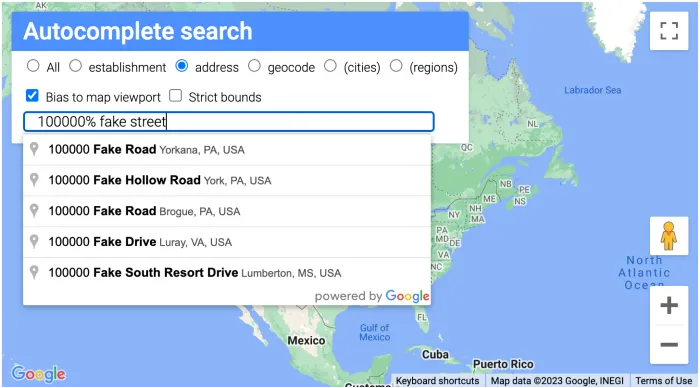
When the autocomplete suggestions are this far off, validation can become impossible.
Questionable Future
Google regularly discontinues promising products when times are good and are even more likely to cut products during layoffs and economic downturns. AngularJS, Google Reader,
Grasshopper, Google Code, Google Search Appliance, App Maker, Google Maps Engine, and Google Maps Coordinate are just a few of the hundreds of products killed by Google before reaching their true potential, and nobody knows what will be next.
How Smarty's Address Validation API Compares
Smarty | ||
Cost (US) | Free for first 12,000 address validation lookups with monthly $200 credit, then:- 50,000 lookups - $850 -150,000 lookups - $2,380 -499,000 lookups - $7,126 | Free for 1,000 address lookup trial + 250 free monthly lookups.- 50,000 lookups - $494- 150,000 lookups - $743 - 600,000 lookups - $5,388Unlimited plans also available |
Address Parsing Components | Parses Address Line 1 components inconsistently | Separates predirectional and suffix components completely |
Query Speed | 100 QPS | Up To 75,000 QPS |
RDI Data (US) | Not consistent for every address | Consistent |
Bulk Validation | Requires custom coding | Yes: Unlimited via API, CLI, Webform |
Address Autocomplete API Includes Validation | No | Yes: US and International |
API Architectural Styles | REST and gRPC | REST |
CASS Certified | Yes | Yes |
Geocodes | Yes | Yes |
Country/Territory Coverage | 33 (as of article publication) | 240+ |
Attribution Requirement | Strict | None |
Data Analysis | Not permitted | Yes |
Data Storage | 30-Days, Limited | Unlimited with a paid subscription |
3rd Party Compatibility | Yes, but may be limited by terms of use & licencing restrictions | Yes |
Customer Support | StackOverflow, 24-Hour to First Response Policy | Extensive: documentation, fully supported SDKs, phone, email, and chat support |
Because Google's Address Validation API is slow, inconsistent, heavily restricted by its data policy, and requires custom coding for bulk address validation, we recommend using our US Street Address API for most address validation cases.
And, since Google's API currently covers a limited number of international addresses, we recommend our International Address Validation API if you work with countries that aren't available in Google's API. Smarty's tools are even less expensive than their Google alternative, so Smarty is the right choice, even if you're on a tight budget.
Excited to start using Smarty's Address Validation tool? Try our International Address Validation API, US Street Address API, or contact sales.